Flocking simulator
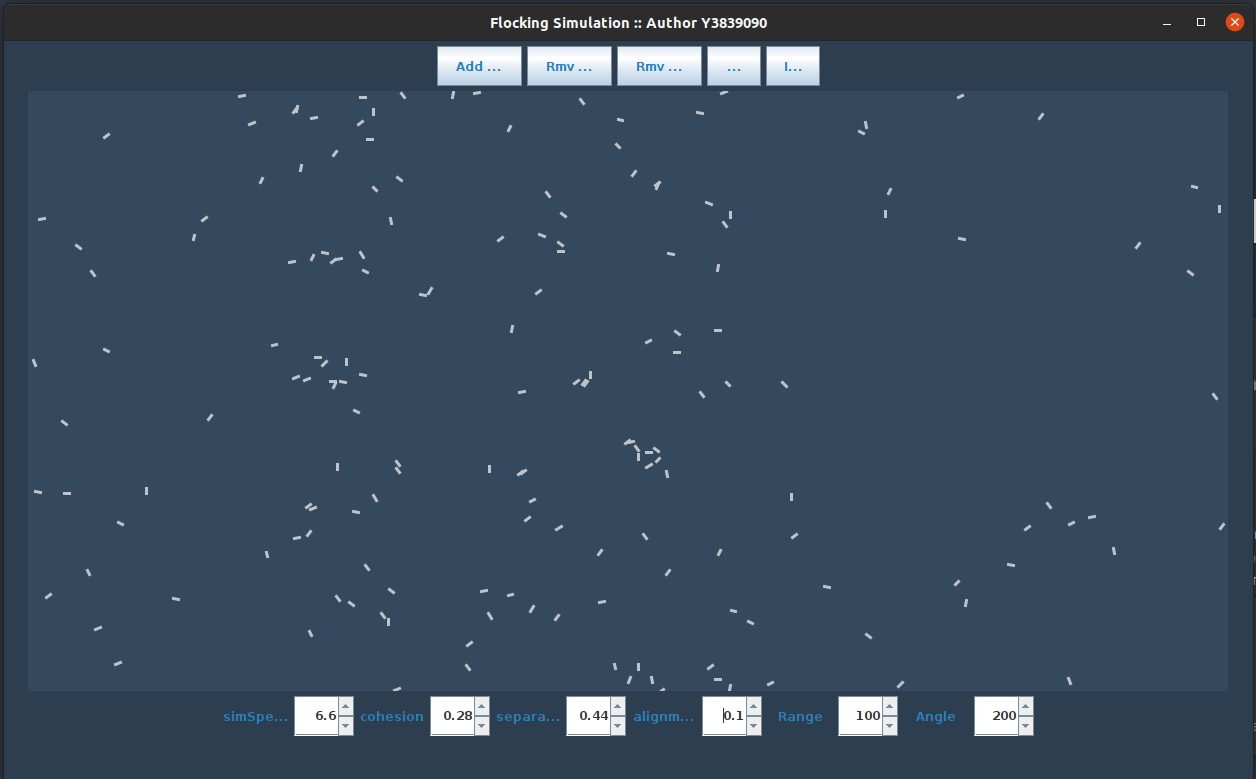
In the first year of my university course, one of my assessments was to create a 'flocking simulator'. Flocking is an emergent behaviour observed in flocks of birds as well as in other groups of animals such as schools of fish. It's characterised by complex group dynamics coming from each individual following simple rules.
Photo by Rhys Kentish / Unsplash
In general, these simple rules are:-
- Don't crash (into others or the ground/seabed/etc)
- Don't Stray too far from the pack
- Follow the pack
Requirements
With this being an assessment, there were some requirements set out for me. I had to:-
- Use Java
- Simulate a flocking behaviour
- Show emergent behaviour
- Allow the number of individuals in the flock to be changed
- Use ObjectOrintated programming
- Have well-structured code
Brainstorming the design
Flocking
In the last lecture before our assignment, our lecturer had told us about the 'boids' method of flocking and advised us to use it. I did some further research on this method to find out more. The best information I found was from the inventor of the boids method, Craig Reynolds. His write up on boids is here[http://www.red3d.com/cwr/boids/] explains the idea and, his paper here[http://www.cs.toronto.edu/~dt/siggraph97-course/cwr87/] gives some more technical information.
UI
I brainstormed about what items the UI should include and, I came up with the following.
- The simulation should be 2D as I had no experience working with 3D libraries in java.
- Start/stop simulation button
- Speed up / slow down simulation * Fix frame rate independent of sim speed.
- Allow addition and subtraction of boids.
- Allow changing the Flocking Algorithm
There were also some problems that I had not yet decided upon.
- Do birds wrap around the screen ? or do they collide with the edges?
- Should there be some randomness in how each individual behaves?
Expected Classes
I then did the same for the basic code and decided upon a class structure and decided I would probably need the following classes:
- Vector - will store a point in 2D space or a velocity in 2D
- Transform - will hold speed, position, velocity Vectors as well as angle and angular velocity floats
- Boid - an individual in the flock
- Simulation - will run updates on all the boids and manage the data structure for the flock
- GraphicsLayer - will be responsible for rendering the Simulation
- UI - will manage the JPanel UI.
Implementation
The assessment gave me the option to use a pre-compiled canvas class. The class managed an instance of JPanel and had a few methods for drawing lines. It was designed for (Turtle Graphics)[https://en.wikipedia.org/wiki/Turtle_graphics] which meant it was unnecessarily restrictive and not appropriate for the task at hand. It was offered to us as we had used it in our last lab and so we were familiar with its interface. I decided not to use this as I thought using JPanel directly would be simpler and more powerful.
I created a similar class from scratch and tailored the methods to my situation. This allowed me to render images instead of just lines for my birds. Which would have been impossible using the supplied canvas class.
My final class structure was more or less the same as what I expected. I did end up adding an extra class, called triggers, to deal with concurrent modification issues. The issues arose because the main thread and the UI event threads were simultaneously accessing/changing the simulation variables controlled by the spinners. I also added the main class that runs the simulation and render loops.
I found the math behind the boid system to be one of the harder parts of the assignment. It took me a couple of tries to get the conversion between the boids local space and the screen space correct. I also had trouble with getting the rendered image to match up exactly with the simulation. The rendered images of the boids are still slightly offset from the boids centre in the final product.
UI
I kept the UI quite simple and attempted a 'flat' design. Although, occasionally, there are still borders and shadows on the buttons because the styling is inconsistent between OS's (so much for java being platform-independent).
I developed the App colour scheme using (flatuicolors)[http://flatuicolors.com/], which helped the UI look consistent.
I changed to using spinners for the flocking 'constants' and simulation speed because they take up less room than the sliders I had used originally.
Boid art
With my custom rendering class, I was able to render images onto the canvas. So I decided to draw a pixel art bird. I am not artistic, so I stuck to a simple bird design (again using flatuicolors [http://flatuicolors.com/]).
I had some issues with the pivot point and scale of the boid image. I didn't manage to completely fix these, but I did manage to reduce them. This means that the boids rotate a little off centre.
JavaDoc
I decided that I wanted to use JavaDoc to make implementation documentation. This meant I had to learn how to use JavaDoc to write correctly formatted comments. I commented on every class I made with these comments and then built the JavaDoc HTML from the command line. I then supplied this along with my written report and code for the project.
Finished Product
Youtube video
Get the Jar
You can download the jar
here
Marking/Feedback
because this was an assessed project, my app got marked and graded.
I received 84% which is a 1st* (starred first).
The feedback I received was positive in general. But as always, the marker suggested a few things that I could have done to improve the app.
- I didn't use any interfaces in my java code.
This was one of the language features the markers were looking for me to use, to show that I had a good understanding of object-oriented programming. - My code wasn't polymorphic.
This is related to the last point about a lack of interfaces. I didn't feel the need to use them in such a simple program. - The marker suggested that the program would have benefited from a way to add many boids at once.
I completely agree with this/ A button to add -say- ten boids at once would have been great. - more images, and better descriptions for those images
- could have mentioned possible further work